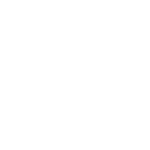
Update 25th of Nov, 2016
Recently I’ve restarted my technical blog under https://kamilgrzegorczyk.com where you can find my newest articles about the development and everything around. I will be posting new tutorials mostly there but I plan to cross-post them also on this website.
A bit of theory
In my last project I tried to emphasize sites’ performance optimization – so I sat down and tried to analyze the impact of various plugins. I was analyzing the output of debug bar and the number of queries, I have also checked the source of particular pages to see what files are being loaded by 3rd party plugins.
Then I came across Cart66 plugin – the plugin which we have used in various previous projects for e-commerce functionality I never was a fan of how this plugin was developed – mainly because of lack of optimization, very slight number of hooks and very limited customization options for advanced devs (overwriting already defined styles is not good way to customize things). Nevertheless it was doing its job – our clients were selling just few items so they weren’t expecting any “magento-like” functionality.
But lets move to the topic – performance – Cart66 was firing even 50-60 queries on pages which were not store pages. How ridiculous is that? It was also embeding CSS and JS files on every WP page . That is why I have decided to limit how the plugin was loaded.
There are mainly 2 ways of disabling the plugins:
- Disabling scripts and styles by using wp_dequeue_style() and wp_dequeue_script( ) functions.
- Disabling the plugin totally by using ‘option_active_plugins’ hook.
First option works flawlessly if we want just to disable styles or scripts from particular pages (for example home page). Unfortunately, if we want to be sure that plugins functions are not fired, that the plugin is not making any unnecessary queries we have to disable it totally.
The code
Lets make few assumptions:
- we know the sites’ structure (page slugs, URLs),
- we are pretty sure of how pages are named and structured (in my case all store pages are children of page named ‘store’),
- we know where the plugin is used on our page,
- we know all downsides of disabling the plugin,
- our usage case is very simple.
As ‘option_active_plugins’ is fired before any plugin is loaded we need to drop our code down into mu-plugins directory (http://codex.wordpress.org/Must_Use_Plugins). Please also remember that those plugins are run before the main query is initialized – that is why we don’t have any access to many functionalities, especially conditional tags which will always return false.
/*
Plugin Name: Cart66 remover
Plugin URI: http://www.lowgravity.pl
Description: Removes cart66 plugin from pages which are not store pages
Author: Kamil Grzegorczyk
Version: 1.0
Author URI: http://www.lowgravity.pl
*/
add_filter( 'option_active_plugins', 'lg_disable_cart66_plugin' );
function lg_disable_cart66_plugin($plugins){
if(strpos($_SERVER['REQUEST_URI'], '/store/') === FALSE AND strpos($_SERVER['REQUEST_URI'], '/wp-admin/') === FALSE) {
$key = array_search( 'cart66/cart66.php' , $plugins );
if ( false !== $key ) {
unset( $plugins[$key] );
}
}
return $plugins;
}
I know that the code is not something “super-extra-fancy”, but it does its job – it excludes Cart66 from being run on all other pages than “e-commerce” ones. I have shaved over 50 queries and “total query time” was cut down by over a half.
We all know that WordPress is a big, complicated environment with many relationships, files, functionalities so If you know any caveats of this approach please let me know in the comments 😉