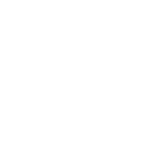
Today I would like to show you how to easily build your own plugin which will manage UI for PODS framework. As Pods is considered a plugin for more experienced users I would consider my tutorial a bit advanced too.
I am a fan of building static classes which can be easily maintained and extended whenever we need it. Why static class? Well – in most cases we will not need fully bloated object approach and multiple object instances so static class is ideal pick for clean and readable code. This is my way of writing functionalities for WordPress – not only the ones based on PODS but for all functionalities in general. There are many other, better or worse, possible ways of handling that – the choice depends on your preferences, experience and situation.
Preparation
First of all please install Pods plugin. Then please import this package so you will have the same basic pods setup as me.
For some reason import is not working as expected and you need to go to books pod setup and select correct relationship for book_author (related to authors) and book_genre (related to genres).
Lets start working.
In my solution we will be building a plugin so this is regular approach as You can find it in WordPress Codex – http://codex.wordpress.org/Writing_a_Plugin. Please refer back to this document if you need more explanation.
First of all we have to create two directories like:
- /wp-content/plugins/lg-pods-plugin/
- /wp-content/plugins/lg-pods-plugin/class/
The next step is to place an index.php file in there:
/*
Plugin Name: Simple PODS framework UI management plugin
Plugin URI: http://www.lowgravity.pl
Description: Manages PODS UI functionalities
Author: Kamil Grzegorczyk
Version: 1.0
Author URI: http://api.lowgravity.pl
*/
// add custom class
require_once('class/LGPodsAdmin.php');
//initialize plugin
add_action('init', array('LGPodsAdmin','initialize_plugin'));
Working with our class
Now there is time for a little bit more advanced things. Please go to class sub-folder and create LGPodsAdmin.php file:
Class LGPodsAdmin {
//what permissions are needed to access pages
const PERMISSIONS = 'manage_options';
//Slug for main page object
const MENU_SLUG = 'lg_pods';
public static function initialize_plugin() {
//initializing menus
add_action('admin_menu', array('LGPodsAdmin','initialize_admin_area'));
//doing some other various stuff
}
public static function initialize_admin_area () {
//set Your icon URL here
$icon = '';
//adding page object -> http://codex.wordpress.org/Function_Reference/add_object_page
add_object_page('LowGravity Pods', 'LG Pods', self::PERMISSIONS, self::MENU_SLUG, array('LGPodsAdmin', 'display_info_page'), $icon);
//in order to not duplicate top menu - first child menu have the same slug as parent
//http://codex.wordpress.org/Function_Reference/add_submenu_page
add_submenu_page(self::MENU_SLUG, 'General Information', 'General Information', self::PERMISSIONS, self::MENU_SLUG, array('LGPodsAdmin', 'display_info_page'));
//genres submenu
add_submenu_page(self::MENU_SLUG, 'Genres', 'Genres', self::PERMISSIONS, 'lg-genres', array('LGPodsAdmin', 'display_genres_page'));
//authors submenu
add_submenu_page(self::MENU_SLUG, 'Authors', 'Authors', self::PERMISSIONS, 'lg-authors', array('LGPodsAdmin', 'display_authors_page'));
//books submenu
add_submenu_page(self::MENU_SLUG, 'Books', 'Books', self::PERMISSIONS, 'lg-books', array('LGPodsAdmin', 'display_books_page'));
}
public static function display_info_page() {
//You can do various stuff here - just an example
echo '<h2>General guidelines</h2>
<p>You can display various informations in here like plugin usage guidelines, copyrights or even add few options to manage - its up to You</p>';
}
public static function display_genres_page() {
//initialize pods
$object = pods('genres');
//for this pod type we will use all available fields
$fields = array();
foreach($object->fields as $field => $data) {
$fields[$field] = array('label' => $data['label']);
}
//adding few basic parameters
$object->ui = array(
'item' => 'genre',
'items' => 'genres',
'fields' => array(
'add' => $fields,
'edit' => $fields,
'duplicate' => $fields,
'manage' => $fields,
),
);
//pass parameters
pods_ui($object);
}
public static function display_authors_page() {
//initialize pods
$object = pods('authors');
//for this pod type we will also use all available fields
$fields = array();
foreach($object->fields as $field => $data) {
$fields[$field] = array('label' => $data['label']);
}
//adding few basic parameters
$object->ui = array(
'item' => 'author',
'items' => 'authors',
'fields' => array(
'add' => $fields,
'edit' => $fields,
'duplicate' => $fields,
'manage' => $fields,
),
);
//pass parameters
pods_ui($object);
}
public static function display_books_page() {
$object = pods('books');
//simple dynamic description functionality - just an idea
$stock_desc = '';
if(isset($_GET['action']) AND isset($_GET['id']) AND $_GET['action'] == 'edit') {
$stock_desc = 'According to ERP software currently we have <strong>'. self::get_erp_stock($_GET['id']) .'</strong> books on stock';
}
//fields on add / edit screen
$fields = array(
'name',
'permalink',
'book_author',
'book_genre',
'stock' => array ('description' => $stock_desc),
);
//fields for manage screen
$manage_fields = array(
'name',
'book_author',
'book_genre',
'stock',
);
//adding few basic parameters
$object->ui = array(
'item' => 'book',
'items' => 'books',
'fields' => array(
'add' => $fields,
'edit' => $fields,
'duplicate' => $fields,
'manage' => $manage_fields,
),
//fields we want to filter
'filters' => array(
'book_author', 'book_genre', 'created'
),
//we need to enable enhanced filters
'filters_enhanced' => true,
//views are not working fully yet but lets implement them just to give you basic idea what they can be used for
'views' => array(
'newest' => 'Show recent ones',
'no-stock' => 'Out of stock',
)
);
pods_ui($object);
}
public static function get_erp_stock($book_id) {
//some fake function which "connects" to external source of data and gets the quantity for us
return rand(0,100);
}
}
Unfortunately, due to time constraints I cannot go through the code line by line. I also think that this tutorial should give you an idea how you can use Pods UI and WordPress plugins API to build your functionalities, not necessarily being a copy-paste resource. I have tried to comment code a lot though so you should very easily get a grasp of whats going on.
I have also included a small bonus – a fake function which loads data from external source and creates dynamic description for stock field on edit screen. That is maybe not the nicest example and usage case of how you can build dynamic UI but this should also give you a feel of what is possible when building UI.
This is just a base, simple setup but its easily extendable – I have plugins with 6-8 classes, each working on different aspects of the site, providing various methods.
That is all for today – if you have any comments or questions – just drop me a line.