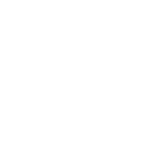
A bit of pods bulk actions history
One of my previous visitors asked for examples showing the usage of bulk actions. The lack of this functionality was one of the main issues of pods in version 1.x. Many of my clients were asking me for a solution to better manage hundreds (or even thousands) of records they had in their DBs. Now we have bulk actions bundled with version 2.2 and we can use it to enhance our UI for better user experience.
Sidenote: have you seen my pods framework code examples on github?
Let’s move on
Enough history – lets move to “here and now” and start the coding. In one of my previous tutorials about pods framework UI I have shown you how to build a simple UI plugin to manage authors and their books. Today I would like extend this plugin to give user a possibility to use bulk actions.
We can use the package from the previous tutorial so please download it here if you don’t have it yet. After you download it, please perform one change in our data structure – remove “required” parameter from the stock field in books pod. Otherwise if we provide 0(zero) then we will receive a validation error and our bulk action will fail.
Let’s start with something really, really simple. At the moment we are still on pods 2.2 version (2.3 is coming soon) and the plugin comes with one default bulk action – delete. In order to enable this function you simply need to pass an array to your pods UI parameters like this:
'actions_bulk' => array(
'delete' => array(
'label' => 'Delete',
),
)
This short snippet is all that you need to enable built-in delete action. But what should we do if we want to add our own function?
First we need to know what our function should do. The easiest example which came to my mind was to clear book stocks to 0 (zero). That should be a good example of how to add bulk action support to your plugin.
First of all we need to add another array to bulk actions parameter specifying:
- the label used in bulk actions dropdown,
- name of our function which we want to call for each selected item.
'actions_bulk' => array(
//adds custom function for our own bulk action - empty books' stock
'zero-me' => array(
'label' => 'Empty stock',
'callback' => 'empty_stock'
),
)
Next thing is to build our function. Unfortunately – as in previous tutorials I will not dissect the code and explain it line by line. Of course everything will be commented as always.
Few things to remember:
- our function takes pods $obj as parameter,
- $obj->bulk contains all IDs selected by user
- after successful action we need to redirect our user back to manage screen and display him a message,
- pods_redirect redirects us back to our action_bulk callback function so we can finish our process and display final message.
function empty_stock($obj) {
//checks if parameter exists
//if yes - it means that we successfully updated data entries and we can display message to the user
//if no - it means that we are running function for the first time
if ( ! isset( $_GET['updated_bulk'] )) {
//$obj->bulk contains all IDs selected by user
$ids = $obj->bulk;
//if we successfully change our items we need to redirect user afterwards
//false by default
$success = false;
//lets count how many items were affected by our function
$items_affected = 0;
if(!empty( $ids )) {
foreach( $ids as $pod_id ) {
//sanitizing ID
$pod_id = pods_absint( $pod_id );
//if the ID is empty - jump out of the current loop iteration
if(empty($pod_id)) {
continue;
}
//getting our pod data entry
$book_pod = pods( 'books', $pod_id );
//setting the stock to desired number
$book_pod->save( 'stock', 0 );
//we have successfully changed item ( so we need to redirect later )
$success = true;
//increase the number of updated items
$items_affected++;
}
}
//checking if our bulk action completed successfully
if ( $success ) {
//if yes - redirect our user to manage page
//this redirect us again to our bulk action handler
pods_redirect( pods_var_update( array( 'action_bulk' => 'zero-me', 'updated_bulk' => $items_affected ), array( 'page', 'lang', 'action', 'id' ) ) );
} else {
//otherwise display an error
$obj->error( __( "<strong>Error:</strong> Cannot update stock.", 'localization-domain' ) );
}
} else { //if our get parameter was set we can display 'success' message
$obj->message( __( "<strong>Success!</strong> We have successfully updated stock numbers for <strong>". $_GET['updated_bulk'] ."</strong> entry(-es).", 'localization-domain' ) );
unset( $_GET[ 'updated_bulk' ] );
}
//clean up
$obj->action_bulk = false;
unset( $_GET[ 'action_bulk' ] );
//show manage screen
$obj->manage();
}