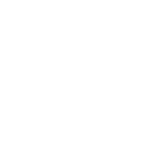
In the third part of my series about PodsCMS 2.x I would like to write a little about new action hooks which were introduced recently. Previously we used a small number of helper functions which were a bit limited when it comes to possible usage cases, their debug and development wasn’t too clear also. Now the things are different.
In 2.x the amount of events where we can hook in has greatly increased in comparison to PodsCMS 1.x. Another good news is that hooks are duplicated for each action – first hook is fired for all types of pods, second one is fired just for particular type of pod – which gives us a lot of possible usage scenarios. We can target our functions to all pods or maybe just for the ones which are freshly created and belong to particular pod type.
!!! Please remember – all hook names are prefixed with ‘pods_{$scope}_ ‘ string so we have use for example “pods_api_pre_save_pod_item” to access our filter !!!
Pod pre-save (scope = api)
Those hooks are called before we save our pod data. We can use them to do some calculations, set values for hidden fields or validate data. They are also very useful if we want to fire specific functions depending on value changes (increase, decrease, relationship changes).
- pre_save_pod_item (ex. pods_api_pre_save_pod_item)
- pre_save_pod_item_{podname} (ex. pods_api_pre_save_pod_item_books)
- pre_create_pod_item (ex. pods_api_pre_create_pod_item)
- pre_create_pod_item_{podname} (ex. pods_api_pre_create_pod_item_books)
- pre_edit_pod_item (ex. pods_api_pre_edit_pod_item)
- pre_edit_pod_item_{podname} (ex. pods_api_pre_edit_pod_item_books)
//general hook
$hooked = $this->do_hook( 'pre_save_pod_item', compact( $pieces ), $is_new_item );
$hooked = $this->do_hook( "pre_save_pod_item_{$params->pod}", compact( $pieces ), $is_new_item );
// if we are creating new item
$hooked = $this->do_hook( 'pre_create_pod_item', compact( $pieces ) );
$hooked = $this->do_hook( "pre_create_pod_item_{$params->pod}", compact( $pieces ) );
//if we are editing existin item
$hooked = $this->do_hook( 'pre_edit_pod_item', compact( $pieces ) );
$hooked = $this->do_hook( "pre_edit_pod_item_{$params->pod}", compact( $pieces ) );
Pod post-save (scope = api)
Those hooks are called after we save our pod item. We can use it for various tasks like email notifications for example. I am using them very heavily, mostly for statistical purposes.
- post_save_pod_item
- post_save_pod_item_{podname}
- post_create_pod_item
- post_create_pod_item_{podname}
- post_edit_pod_item
- post_edit_pod_item_{podname}
//general hook
$this->do_hook( 'post_save_pod_item', $pieces, $is_new_item );
$this->do_hook( "post_save_pod_item_{$params->pod}", $pieces, $is_new_item );
//if we just created new pod item
$this->do_hook( 'post_create_pod_item', $pieces );
$this->do_hook( "post_create_pod_item_{$params->pod}", $pieces );
//if it was an edit
$this->do_hook( 'post_edit_pod_item', $pieces );
$this->do_hook( "post_edit_pod_item_{$params->pod}", $pieces );
Pod pre-delete (scope = api)
Those actions are performed just right before pod item is being deleted.
- pre_delete_pod_item
- pre_delete_pod_item_{podname}
$this->do_hook( 'pre_delete_pod_item', $params, $pod );
$this->do_hook( "pre_delete_pod_item_{$params->pod}", $params, $pod );
Pod post-delete (scope = api)
Finally, post delete actions are very useful for any update scripts, cleaning functions (similar to post-save ones).
- post_delete_pod_item
- post_delete_pod_item_{podname}
$this->do_hook( 'post_delete_pod_item', $params, $pod );
$this->do_hook( "post_delete_pod_item_{$params->pod}", $params, $pod );
Development with easy debugging and sample example
As I mentioned in one of previous articles debugging pod item helpers in podsCMS 1.x was a hard thing mainly because pods data was saved using AJAX calls. Now pods are saved in similar way as WordPress posts – using post-redirect-get method.
Why am I writing about it? Well that’s very important for us because hooks are fired before redirect call is made and all data is not available for us – so simply relying on var_dump() or WP_DEBUG will not work here.
Fortunately this is not a problem and we have various possibilities to solve that problem. Please let me present one way of achieving this task.
I always put pods functionality in separate plugin to have an easy possibility to use pods functionalities on various sites. For our purposes lets just assume that we would like to do it on a theme level.
1. Create file podsDebugger.php in your themes main directory
class podsDebugger {
//display debug data in wordpress admin footer
public static function initialize() {
add_action('admin_footer', array('podsDebugger', 'display_debug_data'));
}
//function saving debug data to file
public static function log_debug_data($data) {
//create small file which will hold our data
$handle = fopen(ABSPATH . '/podsdebug.log', 'a+');
//write our data to that file
fwrite($handle, '<pre>' . $data . '</pre>');
fclose($handle);
}
//function displaying debug data
public static function display_debug_data() {
//echo file contants
echo $dbg = file_get_contents(ABSPATH . '/podsdebug.log');
// and erase file afterwards
$handle = fopen(ABSPATH . '/debug.log', 'w');
fclose($handle);
}
}
2. Open functions.php and add at the bottom
inlcude_once(TEMPLATEPATH . '/podsDebugger.php');
add_action('init', array('podsDebugger','initialize'));
Please change TEMPLATEPATH to STYLESHEETPATH if You are using child theme.
3. Now we are ready to develop and debug our hook functions. Open functions.php once again and add:
add_filter('pods_api_pre_save_pod_item', 'our_pre_save_function', 10, 2);
function our_pre_save_function($pieces, $is_new_item) {
podsDebugger::log_debug_data(print_r($pieces, TRUE));
return $pieces;
}
Now You should see nice array of variables which You can use.
And what now? Well that’s up to you 🙂